Vue
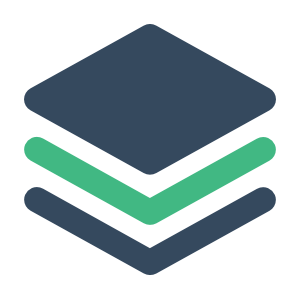
Grid.js has a native Vue wrapper which can be used to create a Grid.js instance within a Vue app. Use the gridjs-vue package to integrate your Vue app with Grid.js.
Install
npm install gridjs-vue
Component Registration
Local Registration
<script>
import Grid from 'gridjs-vue'
export default {
components: {
Grid
}
}
</script>
Global Registration
In main.js
or wherever you specify your global components:
import { GridGlobal } from 'gridjs-vue'
Vue.use(GridGlobal)
Usage
Pass cols
(an array of column headers) and either rows
, from
, or server
as a data source to the component. Everything else is optional.
Refer to Grid.js documentation for specific configuration options.
Basic Example
<template>
<grid :cols="cols" :rows="rows"></grid>
</template>
<script>
import Grid from 'gridjs-vue'
export default {
name: 'Cars',
components: {
Grid
},
data() {
return {
cols: ['Make', 'Model', 'Year', 'Color'],
rows: [
['Ford', 'Fusion', '2011', 'Silver'],
['Chevrolet', 'Cruz', '2018', 'White']
]
}
}
}
</script>
Default Options
{
"autoWidth": true,
"cols": [""],
"from": undefined,
"language": undefined,
"pagination": false,
"rows": undefined,
"search": false,
"server": undefined,
"sort": false,
"theme": "mermaid",
"width": "100%"
}
Extended Options
<template>
<grid
:auto-width="autoWidth"
:cols="cols"
:from="from"
:language="language"
:pagination="pagination"
:rows="rows"
:search="search"
:server="server"
:sort="sort"
:width="width"
></grid>
</template>
<script>
import Grid from 'gridjs-vue'
export default {
name: 'MyTable',
components: {
Grid
},
data() {
return {
// REQUIRED:
// An array containing strings of column headers
cols: ['col 1', 'col 2'],
// AND EITHER an array containing row data
rows: [
['row 1 col 1', 'row 1 col 2'],
['row 2 col 1', 'row 2 col 2']
],
// OR a string of an HTML table selector to import
from: '.my-element'
// OR a server settings object
server() ({
url: 'https://api.com/search?q=my%20query',
then: res => res.data.map(col => [col1.data, col2.data]),
handle: res => res.status === 404
? { data: [] } : res.ok
? res.json() : new Error('Something went wrong')
}),
// OPTIONAL:
// Boolean to automatically set table width
autoWidth: true / false,
// Localization dictionary object
language: {},
// Boolean or pagination settings object
pagination: true / false || {},
// Boolean or search settings object
search: true / false || {},
// Boolean or sort settings object
sort: true / false || {},
// String with name of theme or 'none' to disable
theme: 'mermaid',
// String with css width value
width: '100%',
}
}
}
</script>